I meant to write this blog a long time ago. I always wanted to deep-dive into Intune to assign Microsoft Store Applications via Powershell.
We all struggled with the Microsoft Store applications and assigning them “one by one” through the portal. Even though (almost) every assignment was the same: “Unassign for All Users/All Devices”. Of course, you would want to assign the Company Portal app and maybe another application. But, the general idea is that you would like to assign all Store Apps faster than clicking through the portal.
In addition, Microsoft planned to deprecate the Microsoft Store for Business in 2023. Nevertheless, I share my methods with you to do this via Powershell.
Prerequisites
You need a Powershell Module called “MSAL.PS”.
Install it using the code below:
#Checking for correct modules and installing them if needed $InstalledModules = Get-InstalledModule $Module_Name = "MSAL.PS" If ($InstalledModules.name -notcontains $Module_Name) { Write-Host "Installing module $Module_Name" Install-Module $Module_Name -Force } Else { Write-Host "$Module_Name Module already installed" } #Importing Module Write-Host "Importing Module $Module_Name" Import-Module $Module_Name
Assigning Store Application via Powershell
We are using Microsoft Graph to get the information and assign groups to the applications. So, firstly we need to log on to Microsoft Graph.
In Azure AD, there is an Enterprise Application called “Intune Powershell”. We leverage this Application to log on. Therefore, the application id in the code below is the same for each Microsoft 365 tenant.
We do so by using the following code:
$AuthToken = Get-MsalToken -ClientId d1ddf0e4-d672-4dae-b554-9d5bdfd93547 -RedirectUri "urn:ietf:wg:oauth:2.0:oob" -Interactive
Log in with a user account that has the appropriate permissions for Microsoft Intune.
After that, run $AuthToken to view the token:

Furthermore, if you want to know which permissions your token has, run $Authtoken.Scopes:

Next, we need to get the information about the Microsoft Store Apps within our Intune tenant. Use the following code to get the information:
## Get Microsoft Store Applications $Url = 'https://graph.microsoft.com/beta/deviceAppManagement/mobileApps?$filter=(isof(%27microsoft.graph.microsoftStoreForBusinessApp%27))' $StoreApplications = (Invoke-RestMethod -Headers @{Authorization = "Bearer $($AuthToken.AccessToken)" } -Uri $Url -Method GET).Value | Select-Object publisher,DisplayName, id, isAssigned
This is the output:
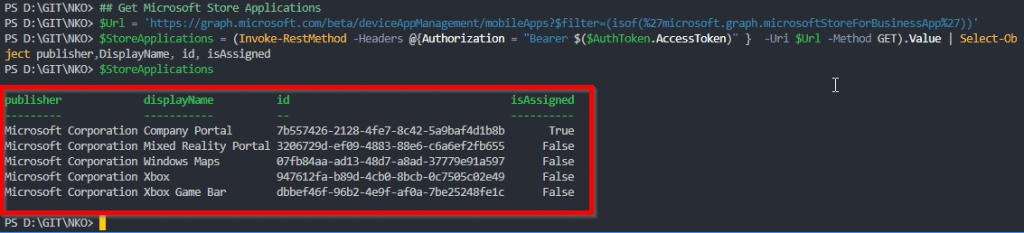
Furthermore, If you want all the details. Remove ” | Select-Object publisher,DisplayName, id, isAssigned”
Lastly, we need to assign the application. You can do so by using the following code:
I have used the Company Portal app as an example. The $ApplicationID is the application ID grabbed from the previous output. This is the assignment “Required for All Users”: (I specify the $JSON body for All Devices and Custom Group later)
$ApplicationID = "7b557426-2128-4fe7-8c42-5a9baf4d1b8b" $JSON = @" { "intent": "required", "target": { "@odata.type": "#microsoft.graph.groupAssignmentTarget", "deviceAndAppManagementAssignmentFilterId": null, "deviceAndAppManagementAssignmentFilterType": "none", "groupId": "$AzureADGroupID" }, } "@ $URL = "https://graph.microsoft.com/beta/deviceAppManagement/mobileApps/$ApplicationID/assignments" $AssignApplication = Invoke-RestMethod -Headers @{Authorization = "Bearer $($AuthToken.AccessToken)" } -Uri $URL -Method POST -Body $JSON -ContentType 'application/json'
This is the output:


$JSON Body for All Devices assignment:
$JSON = @" { "intent": "required", "target": { "@odata.type": "#microsoft.graph.allDevicesAssignmentTarget", "deviceAndAppManagementAssignmentFilterId": null, "deviceAndAppManagementAssignmentFilterType": "none" }, } "@
$JSON Body for a custom group:
$AzureADGroupID = "7fbb81e7-aa9a-4c38-9416-808c0b90744b" $JSON = @" { "intent": "required", "target": { "@odata.type": "#microsoft.graph.groupAssignmentTarget", "deviceAndAppManagementAssignmentFilterId": null, "deviceAndAppManagementAssignmentFilterType": "none", "groupId": "$AzureADGroupID" }, } "@
References
Graph Explorer (Used for looking up the correct JSON body’s)
Other posts about Graph:
Create Autopilot Profile via Powershell
Intune Config Backup to Storage Account
Is there an equivalent process for Android/iOS apps?
Hi Ian,
Thanks for your comment. This will likely change in Q2 in 2023, I will update my blog accordingly.
Regards,
Niels
Hi Niels, thanks for this post. Please don’t forget, “This will likely change in Q2 in 2023, I will update my blog accordingly.”
Sincerely,
David
Hi David,
Thanks, I need to look in to this.
Niels